Why Should an SEO Professional Care About Programming?
Although there’s no formal definition of skills and knowledge that an SEO professional should possess, it’s best to understand which technologies being used by a website contribute to technical SEO. If problems arise, you should be able to suggest which technology implementation needs to be initially investigated. A developer or engineer is required to resolve most errors, but there are situations when you can solve smaller and simpler problems. Or provide useful suggestions for quicker troubleshooting.
It’s possible to create a blog post for each facet of technical SEO, but this page’s dedication is to programming languages. The way web pages are constructed has a big impact on SEO, and this begins with HTML tags and CSS properties. Since JavaScript provides interactive features for the front-end, it’s important to have some knowledge of it. And due to the nuances of server-side and client-side renderings, the choice of programming language also impacts SEO.
Wouldn’t it be helpful to know at least a little about the programming languages used for web development? Let’s take a sneak peek at the programming languages used on the internet and read some personal stories about how having some programming skills has helped this SEO professional achieve business goals.
What is “Technical SEO”?
Technical SEO optimizes the website’s architecture to produce the most SEO-friendly web pages. Here are common technical areas that impact the optimization of a website:
- Page loading speeds
- Crawl management (internal link structure and “robots.txt” file)
- Server response codes (200, 3XX, 4XX, and 5XX response codes)
- HTML, CSS, and JavaScript (front-end frameworks)
- Redirects
Programming languages impact all of these items. Some functions can increase the speed of loading pages or make them slower. Search engines have distinct treatments for particular HTML tags. You can program redirects and even change the server response codes. And there’s client-side rendering (the browser sends the HTML syntax to itself) versus server-side rendering (the server sends the HTML syntax to the browser).
Text Editors
A text editor is required for coding. Most operating systems come with basic text editors (usually notepad programs), but intuitive editors exist. Notepad++ is a good example of a smart editor with some features to identify programming syntax. My personal preference is Sublime Text, which has project management features and is capable of providing definitions for built-in functions of certain programming languages. I prefer its syntax formatter. Another popular text editor is VSCode, but since I haven’t used it I can’t provide an opinion. There’s no standard code editor; use the most comfortable one.
Programming Languages of the Internet
This section of the blog post introduces you to the programming languages that run the internet. “HTML and CSS” deserves a long explanation because they’re considered bedrocks of all web pages. The other areas won’t be as lengthy in explanation. “Coding Resources” at the bottom of this blog post provides resources where you can get hands-on with learning programming languages.
HTML and CSS
HTML and CSS are the bedrock of the internet. No web page gets built online without their usage. HTML is the lumber that upholds a website’s walls, while CSS is the paint that colors it. They’re not programming languages. The closest word to use is syntaxes. HTML is a collection of tags; CSS is a collection of properties connected to tags using two attributes within a tag: “class” and “id.”
So what are tags?
<html>
...
</html>
The two tags above are the opening HTML tags for a web page. “<>” begins a tag, and “</>” ends the implementation of a tag. Not all tags need a closing implementation. Usually, tags that imply sections or text formatting have an opening and closing. A list of HTML tags and their usage is available on W3Schools.
The HTML structure of any web page follows the structure below:
<html>
<head>
...
</head>
<body>
...
</body>
</html>
Let’s explain the HTML structure. <head> follows after the opening <html> tag. It’s usually called the “header” section, but it’s also known as the “head” or “metadata” section of a web page. This is where “meta” tags are inserted (if you work in SEO, you’re familiar with that term). <body> follows the closing “header” tag; this area is where all the front-end HTML tags will be rendered. “…” implies that other HTML tags can be inserted within that section.
HTML tags can have what are called “attributes.” These are extra properties inserted within a tag that changes the rendering appearance of particular HTML tags. Each HTML tag contains distinct “attribute” properties, and you can visit the W3Schools website to explore “attributes” for each tag. There are two “attributes” which apply to any HTML tag: “class” and “id.” They connect CSS rules to specific HTML tags.
What is CSS? Like HTML, it’s a syntax language that’s not considered a programming language; it’s a bedrock of the internet. You can create selectors; each selector contains a collection of properties, each with a single value. Each property and value combination is called a declaration.
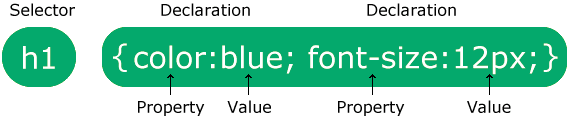
A selector can be any of the following: an HTML tag name, a class name, or an ID name. Class is CSS rules that apply to multiple HTML tags, whereas ID is CSS rules that apply to a single HTML tag (in practice, but some folks mistakenly apply ID rules to multiple tags). In the illustration above, the <h1> tag will have a blue text color with a size of 12px. Here are examples of various CSS rules:
/** HTML tag selector rules **/
h1 {color:blue; font-size:12px;}
/** Class selector rules **/
.class-name {color:blue; font-size:13px;}
/** ID selector rules **/
#id-name {color:blue; font-size:14px;}
/** HTML tag with "class-name" class attribute rules **/
h1.class-name {color:blue; font-size:13px;}
/** HTML tag with "id-name" id attribute rules **/
h1#id-name {color:blue; font-size:14px;}
How do we apply these CSS rules to an HTML tag? If you’re setting up an HTML tag selector rule, there’s nothing you need to do except insert the HTML tag into the document.
<h1>Blue text, Font size 12px</h1>
How do you apply a class or ID selector rule? You use the “class” and “id” attributes, respectively.
<h1 class="class-name">Blue text, Font size 13px</h1>
<h1 id="id-name">Blue text, Font size 14px</h1>
You can also combine the two attributes in a single HTML tag. One caveat is that if the two selectors have the same declarations, the ID attribute will override the class attribute when combined. In the example below, “id-name” CSS rules will override “class-name” CSS rules.
<h1 class="class-name" id="id-name">Blue text, Font size 14px</h1>
However, before you can use CSS on a page, you must declare them. There are three ways of doing a declaration. The first is by using a “meta” tag to create a link to what’s called a “stylesheet” document that contains CSS rules (usually ending with “.css” as the file extension). The second way is using what called “internal” rules, utilizing the <style></style> tags to insert CSS rules into the web page. The third way is called “inline,” which uses the “style” HTML attribute to insert rules. Following the example of the <h1> tag displayed earlier as an example, here are more examples showing ways to declare them.
<!-- In case you're wondering, this is how to insert comments into HTML code -->
<!-- Everything encapsulated within this syntax is invisible on the screen -->
<!-- But it is visible if someone views the Page Source -->
<!-- stylesheet declaring -->
<link rel="stylesheet" href="url_of_some_file.css">
<!-- internal declaring -->
<style>
h1 {color:blue; font-size:12px;}
.class-name {color:blue; font-size:12px;}
#id-name {color:blue; font-size:12px;}
h1.class-name {color:blue; font-size:13px;}
h1#id-name {color:blue; font-size:14px;}
</style>
<!-- inline declaring -->
<!-- note: I'm only showing a single CSS rule declaration -->
<h1 style="color:blue; font-size:12px;">Blue text, Font size 12px</h1>
There are LOTS of CSS properties in existence, and some of them are complicated. You can use W3Schools CSS Syntax page as a starting point for exploring different CSS properties at your disposal.
Earlier, I mentioned “meta” HTML tags that fit in the <head> section. Some are specific to SEO, and familiarity with them is very handy. Here are the “meta” tags you should know about:
<title>...</title>
<meta name="description" content="...">
<meta name="robots" content="...">
<link rel="canonical" href="...">
<meta prop="og:title" content="...">
<meta prop="og:description" content="...">
<meta prop="og:url" content="...">
<meta prop="og:image" content="...">
<meta http-equiv="Content-Type" content="...; charset=...">
<meta charset="...">
<meta name="viewport" content="width=device-width, initial-scale=1">
Explaining each “meta” tag would take far more space to write about. ContentKing has written a useful meta tag guide that helps you understand the purpose of these “meta” HTML tags and how to maximize them. They also show additional “meta” tags that I haven’t included here but may still be useful.
JavaScript
If HTML and CSS are the walls and painting of a website, then JavaScript represents the novelty decor items. Dropdown menus, carousels, accordions, and other interactive features on a web page are possible due to JavaScript.
Using JavaScript on a page involves the <script> tag. You can insert code in between the opening and closing tags. Or, you can create an external file that contains JavaScript code and then link it using the “src” attribute.
<script type="text/javascript">
//type code directly on the page
...
</script>
<script type="text/javascript" src="some_url_to_file.js"></script>
The most common JavaScript you’ll encounter is “Vanilla JS” or “Native JavaScript.” They both refer to code that isn’t dependent on a framework (and there are LOTS of frameworks). Here are some most-used frameworks you’ll likely encounter (this list isn’t exhaustive):
- jQuery
- React
- Angular
- Next.js
- Backbone
- Node.js
- Vue
You can read this article from Hackr.io to learn about the most popular frameworks being used today. For a good book about JavaScript programming, I recommend you read JavaScript & jQuery from Wiley publishing.
There are two aspects of JavaScript you should know about: client-side and server-side renderings. With client-side rendering, the browser sends the HTML syntax to itself. With server-side rendering, the web server sends the HTML syntax to the browser. By default, JavaScript is a client-side programming language. This means that search engine crawlers won’t crawl JS-rendered HTML output easily. Crawlers work best with server-side rendering; JavaScript may need special handling and careful planning to make it SEO-friendly. I plan to write a future blog post about this topic, but for now, know that default JavaScript doesn’t render SEO-friendly HTML. There are ways to accomplish server-side rendering, but it involves backend development and server configurations to make it happen. You can Google for JavaScript frameworks that accomplish server-side rendering. React, a popular framework, is capable of SSR with some special configurations.
Another aspect to know about JavaScript is the concept of “eventlisteners.” Clicking, scrolling, typing in a form, submitting a form, and other actions are events that happen on a page. You can setup “listeners” so that when these events occur, defined functions can be executed. An old-fashioned way of implementing this is by using the “on” HTML attribute. The most popular “on” attributes are onclick and onload. Many others exist, but these two are the most used. Crawlers cannot execute “eventlisteners” nor can they detect them. I provide a story about how this fact can work to your advantage in the “Trenches” section below.
TypeScript
TypeScript is a sort of improvement extension of JavaScript. It’s complicated to explain. The programming language is expected to grow in prominence because it confronts deficiencies in JavaScript (a popular choice among developers). TypeScript’s syntax is very similar to JavaScript; unlike the language, it supports server-side and client-side rendering. I can’t talk much about the programming language since I haven’t used it, but there are websites adopting TypeScript. To learn more about TypeScript, read its main website and then explore popular course websites to find online lessons about the programming language.
PHP
Alongside JavaScript, PHP is one of the earliest programming languages for the internet. Some developers cynically consider PHP controversial and no longer necessary. One could argue that if it weren’t for WordPress, PHP would never make it to versions 7 and 8.
Unlike JavaScript, PHP is a server-side programming language. This means that web pages are rendered by the server, making them more SEO-friendly than client-side rendering languages. Most web hosts make it easy to execute PHP code (largely because they allow WordPress hosting).
There’s a lot to talk about PHP. It’s possible to build many applications with this programming language, and it’s used on thousands of websites. Documentation for PHP can be found on php.net, and you can find tutorials on any course platform.
WordPress
WordPress is a blogging platform, powering 40% of the internet. It’s not a programming language, nor does it provide its coding syntax, but it does feature a distinct framework that savvy developers can bend to their will. There are theme and plugin functions; underneath those are functions for database and data parameters retrieval. All this makes the blogging platform capable of more than just blogging. There are capabilities for e-commerce, learning management systems, directories, large-scale online magazines, and more. WPEngine has a blog post that details the many utilities of the platform. Plugins are the most used method for extending WordPress beyond blogging.
There’s a ton of support on the internet to help people develop WordPress capabilities. WordPress.org has an extensive knowledge base that explains theming and creating plugins; the developer documentation handbook contains extensive documentation about each function in WordPress’s native framework. All of which are programmed with PHP. SEO professionals familiar with basic PHP can navigate the WordPress framework to add SEO-friendly features to WP-powered websites. Title tag changes, taxonomy links, pagination, even CSS styling—all these modifications and more are possible if SEO professionals are willing to use PHP and WordPress functions. I provide some WordPress stories of my own in the “Trenches” section below.
Python
Python is the most popular programming language for the web, and it’s not surprising why that’s the reason. First, the syntax is renowned for being extremely easy to understand. Second, the programming language is flexible—it’s useful for data science and data analysis, web page creation, and artificial intelligence. I even created a blog post that curates Python resources for SEO professionals. The Python framework used for web development, Django, is a server-side framework—meaning it’s useful for creating SEO-friendly pages. I’m currently learning Python and exploring the language, so I can’t showcase or provide any further explanations about this programming language. I can say that it’s worth being aware of Python because SEO professionals may encounter it in the field.
C# and .NET
The C language is one of the oldest programming languages in existence. Although C is not used much, its spin-off—C++—is more utilized for application development. Many web browsers and desktop applications are created with C++. The programming language itself is, er, complicated. There’s much more emphasis on types and other deep programming concepts. Microsoft released its version of the C language called C#—pronounced “C-sharp”—to remove some of the programming complexity in the C language. C# is part of a suite of Microsoft programming languages called .NET. Dotnet is Microsoft’s attempt at creating a cross-platform suite that runs on multiple operating systems and computer hardware. It’s difficult to explain, but know that web pages are coded with C# and the dotnet (.NET) framework. If you ever encounter web pages with the .ASP file extension, know that .NET and most likely C# are the primary framework and programming language. ASP pages are usually highly specialized and most certainly server-side rendered. The C programming language is highly complex, and a software developer is required to make even the simplest change.
Special Mentions
There are other programming languages you might encounter on the internet.
- Rust
- Go
- ColdFusion
ColdFusion is the oldest programming language in the list above. It’s famous for being very secure and lacking vulnerabilities in other programming languages. I’ve only encountered this programming language once on an e-commerce website. ColdFusion is probably reserved for specialized websites, and you’re not likely to encounter an optimized website built with the programming language.
Rust and Go programming languages are based on the C language. Think of them as the C-language for the internet. They’re compared to Python’s execution speed most of the time. You might not encounter Rust and Go much because they’re relatively new and still being adopted by some web developers. I can’t think of a website written in Rust and Go. Nonetheless, being aware of these two programming languages won’t hurt.
Database Query Languages
Most websites use a CMS (content management system), which deploys databases to power their functionalities. Although you’ll never be the person responsible for creating and managing databases, knowing how to navigate a database can be useful. I describe a database story in the “Trenches” section if you’re looking for an example of relevance.
There are three general types of databases you’re likely to encounter in the field: MySQL, PostgreSQL, and NoSQL.
MySQL
MySQL is a lightweight version of SQL (short for “Structured Query Language”), a database system known as a “relational database management system” (or “RDBMS” for short). You can create distinct tables and connect them when necessary. It’s open-source and not as secure as SQL Server, but it’s free to use and comes with most web host plans. WordPress uses MySQL.
I won’t bore you with the differences between MySQL and SQL (it doesn’t matter to you as an SEO professional). What matters is that you know how to run queries on a MySQL database. I won’t bore you with that, but you can read an article about the 10 most common MySQL queries. You’ll find SELECT (the most common), INSERT, DELETE, UPDATE, and JOIN commands. In a PHP environment, you’re likely to be using PHPMYADMIN to interface with the MySQL database. You can test query commands in the interface to see what data is returned before you integrate the commands into your code.
PostgreSQL
PostgreSQL is similar to MySQL with a somewhat minor difference. It’s object-oriented and supports more data types than MySQL. As an SEO professional, this difference is probably minor. Many query commands have the same syntax as MySQL, although some functions vary. I’ve encountered some websites that use PostgreSQL, and that’s why I mention it.
How to Connect to a SQL Database
There are two ways to connect with a SQL database. If you’re in a PHP environment, you can use PHPMYADMIN, which is usually included with your web host plan. Otherwise, a GUI tool can be used. A popular one for MySQL is called MySQL Workbench. You can find similar tools for PostgreSQL databases.
NoSQL
NoSQL databases are probably obvious: they’re database systems that don’t use Structured Query Languages. According to MongoDB (a NoSQL database provider), there are four types of NoSQL databases:
- Document databases
- Key-value databases
- Wide-column stores
- Graph databases
You’ll need specialized software for querying each of these types of databases. SEO professionals don’t need to be aware of the intricacies of each type. Still, they should be aware of the database they might be dealing with if there’s a need to query these databases for information. MongoDB is one of the most popular choices for NoSQL. Their Compass software works similarly in principle to MySQL Workbench, where you can create clusters (“databases” in MySQL) and collections (“tables” in MySQL).
Coding Resources
- Free Code Camp: This is one of the ultimate coding resources for beginners. You’ll find structured tutorials for many programming languages and databases mentioned in this blog post.
- Coursera.org: There’s no shortage of programming courses on this site. You’ll discover online classes from coding professionals and infamous Silicon Valley corporations.
- Stackoverflow: A community for asking and answering programming questions. It’s a good place to go if you’re stuck on a coding problem and have no idea how to solve it.
- JSFiddle: This is a good website for testing HTML, CSS, and JavaScript code.
Programming Stories from the SEO “Trenches”
Never let an opportunity go to waste.
Posters.com
Posters.com was a website purchased by the company that owned AllPosters.com and turned over to the SEO team as a microsite. For years, it laid dormant as a landing page linked to key category pages on the AllPosters. Until I was promoted to the title of “SEO Associate.” First, I installed Google Analytics UTM parameters on every hyperlink that led to the main site (to track which category links generated the most revenue). Second, I created landing pages that ranked for popular poster sizes—a feat that couldn’t be accomplished on the main site due to limitations. Then I migrated the entire site to a WordPress installation. I chose and modified the WP theme myself, using my limited knowledge of PHP and the WordPress framework at the time. After porting internal API code from Poster.de (more on that below), I could scale the creation of product grids and landing pages on Posters.com and rank for more search terms.
Poster.de
Like Posters.com, Poster.de was a website purchased by the company that owned AllPosters.de and turned over to the SEO team. Unlike Posters.com, the microsite functioned and was structured like a full e-commerce site. There were category and other taxonomy pages, a homepage showcasing featured products, and product pages with prices and images—with “Buy Now” CTAs that led to product pages on AllPosters.de. The framework consisted of multiple PHP components that involved 40 files using an older version of PHP (4.7).
One day, Google Search for Germany started to de-rank the AllPosters.de website for the very popular search term poster. After analyzing the situation, I noticed that the search engine was prioritizing homepages using SSL certificates and also contained the keyword multiple times on the homepage. This was a problem because it wouldn’t have been possible to install an SSL certificate plus insert the keyword poster multiple times on the homepage of AllPosters.de (due to limitations). However, the Poster.de homepage didn’t move from its original rank position; eventually, AllPosters.de would drop to Page 2. This meant that Poster.de had a greater chance of at least maintaining a presence for the company on this search term. But given how mobile unfriendly and non-SSL the site was at the time, I knew this advantage wouldn’t last. Since there weren’t PHP developers in the company, and the site was too small for the rest of the organization to care about, I was the only person available to tackle this situation.
I first needed to understand the key pages that generated revenue and traffic for the main site, AllPosters.de. It wasn’t apparent at first how the framework functioned (no documentation existed). There were a bunch of “include” statements of PHP file components. I took an 11×14 piece of paper and mapped which PHP files were “included” and their relationships to other PHP files. After this herculean task was finished, I discovered the framework consisted of 40 files. I installed Google Analytics UTM parameters on links that led back to AllPosters.de, and I discovered which pages generated revenue and combined that with my SEO analysis of key pages. I dug further into the web server administration, figured out the routing scheme, and learned about PHP MySQL functions (this was also my first exposure to MySQL). And I found the internal API code that a previous SEO Associate, Sonny B., programmed in PHP (he left very thorough notes about the API code that I was able to follow).
After all these discoveries, I created a new version of Poster.de. First, I found an ideal HTML template (mobile-friendly and modern looking) and purchased it for less than $30. I replaced certain HTML content with PHP code and successfully reproduced the homepage content of the current site. Then, I recreated the category, taxonomy, and product pages. This all took me a little less than two weeks. When I created all the pages, I showed them to my manager, Mahalingam V. He quickly scheduled a meeting with our VP of International Marketing, who became familiar with the situation and excitingly hopped onboard to support this side project of mine. Then we involved the server administrators in developing an upgrade plan—including the installation of SSL for Poster.de. Two weeks later, just as Poster.de was beginning to decrease in rank for poster, the microsite upgrade was completed. By the third day of the post-upgrade, Poster.de had increased its rank for the search term. My boss later joked that for 10 minutes, we achieved the #1 rank for the keyword—a feat the company was never able to achieve on Google Search in Germany.
I later ported the internal API PHP code previously developed by my ex-colleague, Sonny B. It would eventually land on Posters.com, AllPosters.com Blog, and the International AllPosters Sites (more on that below).
This side project may have also promoted me to “SEO Analyst.”
AllPosters.com Blog
The AllPosters.com Blog was an initiative started by another ex-colleague, David W. It was a blank slate, although the theme and sub-domain were deployed. Since I had the freedom to publish content without brand approval, I commenced writing content around pop culture topics. The experience allowed me to refine my keyword research skills, and I ranked many articles for various search terms. My Star Trek Redshirt article was even cited in a Wikipedia article. Often, I tinkered with the underlying PHP code to accomplish simple stuff (category links, title changes, display appearances, etc.).
But none of those compared to my ultimate achievement, creating a related product grid. Remember how I mentioned porting the internal API code to Posters.com and AllPosters.com Blog from Poster.de? I used the code to create related product grids based on the content of a blog post. Then I added SEO team attribution parameters and generated revenue from product sales (paltry compared to our main site, but an accomplishment nonetheless).
PosterRevolution.com
Poster Revolution was another website purchased by the company. Unlike the others in this list, it was never turned into a microsite. The operators of the site worked as employees and later left the company. Since my employer had no idea how the site functioned, it was decided to keep the domain but host it on a different e-commerce platform. Someone chose BigCommerce, and due to my success with the other microsites, it became my responsibility to build the site. I found a theme, installed it, and made alterations to make a proper design.
An SEO issue quickly arose: since we weren’t able to recreate all the old category and product pages. 301 redirects wouldn’t be feasible at first. We were limited to creating ten categories, a far cry from the nearly 200 categories configured on the old platform. How did I solve this problem?
- This was when I taught myself JavaScript and was reading about Google’s capability for doing limited crawling of JS code. Applying what I read, I learned that I could change META tags. I turned search results pages (which weren’t indexed by default and had a badly formed TITLE tag) into indexable pages with an optimized TITLE tag.
- Using a redirection tool within the platform, I created redirects from old category URLs to the equivalent search results pages.
The result was increased rank and traffic for pages on the new PosterRevolution. If not for another project I switched to, I could’ve fully recovered organic traffic for the site.
I did port the JavaScript code to the main U.S. AllPosters site. The TITLE tag was able to be altered, plus I added text snippets to category pages. A capability that the SEO team craved for many years!
Ketofoodist.com
The website existed for a couple of years before I added features to make it function like a search engine. The main feature was the introduction of a filtering system, and its very first iteration involved the <a> tag. An SEO issue quickly emerged when I noticed my ranking began to fall for most search terms. I immediately surmised that the introduction of the HTML tag created internal link flow problems.
But how else could I trigger the filter action?
I didn’t think to use HTML form actions, or there might’ve been a reason why it wouldn’t work, but the solution that emerged was to force Google to ignore the link. I knew at the time that the search engine couldn’t detect JavaScript “eventlisteners” so I opted to use those instead. Whenever the APPLY button was clicked, it would load a new page with filter parameters. After implementation, my SERP visibility and traffic returned to normal. Problem solved!
International AllPosters Sites
I saved the best story for last! This project combined all the knowledge gained from working on the microsites.
A new CEO joined the company, and one of her first initiatives was to kill four international AllPosters websites (AllPosters.cz, AllPosters.pt, AllPosters.pl, and AllPosters.com.tr). My Director and a group of engineers wanted to save the domains and at least turn them into pages that didn’t require active maintenance from technology teams. They convinced her to save the domains, but the deadline was 1.5 months to enact an initiative—or else lose them forever! I was invited to a meeting, and my Director proposed two solutions: (1) turn them into BigCommerce sites, or (2) take the old Posters.com approach and turn the domains into single-page websites. I disagreed with both approaches.
- BigCommerce was a giant pain to manage, and we’d lose traffic just like with Poster Revolution (these AllPosters sites contained a more complicated URL structure that would’ve been impossible to create 301 redirects due to limitations).
- Turning the domains into landing pages would make us lose the URL structures and TITLE tags that helped the pages rank, resulting in a loss of traffic and negating any benefits of our project.
I proposed a third solution: WordPress. Recreate the sites using an installation of the popular blogging platform. The room fell silent. You could hear the waves from the bay below pounding the Emeryville coastline under the office tower. “Are you sure?” my Director asked. I nodded, but just in case this was a bad idea, I told them I’d research the solution before reaching an official conclusion. During the week, I gathered all the table schemas of the WordPress database. I researched whether I could change the default URL structure of WordPress and if I could import our e-commerce categories into the platform’s database tables. Although I couldn’t find direct answers, from the function documentation I read, my solution seemed more feasible. I gave a resounding, confident “Yes!” as my answer that week.
I began the week after. My Director provided a SQL table export of our current categories. I formatted the output in Excel and then used PHPMYADMIN to import them into the WordPress category tables, overriding the default categories created. Then I found a mobile-friendly theme (these international sites were never originally mobile-friendly), and we even setup SSL certificates beforehand. I ported the internal API code. It took some time to figure out how to alter the URL structure and regex path retrievals, but I eventually managed to replicate the AllPosters URL structure. My previous ex-colleague, Sonny B., extensively documented the SEO scheme of META tags on the main sites (which involved pagination and product ID numbers). I recreated the same schemes on WordPress for the category and product pages. Later, I recreated all the translations by asking my manager for an export of the translation SQL table; any translations not available in the table would rely on Yandex’s free translation API. With all the programming work completed a week before the deadline, the sites were launched, and the DNS records were all pointed to the new WordPress installations for all four websites. There were some hiccups with the original web server being able to handle the traffic. Still, I eventually found a private server offering on Dreamhost that could handle the barrage of web traffic that the sites received.
What were the results? I saved the SERP rankings of the four AllPosters websites, traffic to the domains increased, and revenue grew 10% more than the previous year across the four domains. My manager planned to discuss relaunching PLA (product listing ads) for all four domains due to the project’s success. Sadly, those plans never came to fruition. On a positive note, the lessons I gained from this project carried over to another website I created, ketofoods.info—the first iteration of the keto food search engine which would later evolve into Ketofoodist.com.
What’s the point of bringing up my programming stories from the “trenches?” To show that programming knowledge can save your ass. This blog post focuses on SEO, but any business professional can benefit from knowing how to program. Whether it’s for web development or data analysis or automating a task, or something else. You can add value to your company and your boss and, more importantly, make yourself invaluable with some programming know-how. Keep an eye out for the latest usable programming languages, use the coding resources to teach yourself some coding, and create your own programming stories that you can one day place in a blog post.